Golang Testing Example: An Introduction to Writing Tests
Introduction:
Writing tests is an essential aspect of software development, as it helps ensure the stability and correctness of our code. In Go, the testing framework provides a simple yet powerful set of tools for writing tests. In this article, we will explore an example of how to write tests in Golang using the "testing" package.
## Getting Started with Golang Testing
In Golang, tests are written in separate test files with a "_test" suffix. For example, if we have a package named "math", the corresponding test file would be "math_test.go". Let's consider a simple scenario where we want to test a function that calculates the factorial of a given number.
## The Factorial Function
Firstly, let's define our factorial function:
```go
package math
func Factorial(n int) int {
if n == 0 {
return 1
}
return n * Factorial(n-1)
}
```
## Writing Tests
Now, let's write tests for our factorial function. Create a new test file called "math_test.go" and add the following code:
```go
package math
import "testing"
func TestFactorial(t *testing.T) {
cases := []struct {
input int
expected int
}{
{0, 1},
{1, 1},
{5, 120},
{10, 3628800},
}
for _, c := range cases {
result := Factorial(c.input)
if result != c.expected {
t.Errorf("Factorial(%d) == %d, expected %d", c.input, result, c.expected)
}
}
}
```
In the above code, we define a struct for test cases, which includes the input value and the expected result. We then loop through each test case, calculate the factorial using our function, and compare it with the expected result. If there is a mismatch, we use the `t.Errorf` method to log an error.
## Running Tests
To run the tests for our package, we can use the `go test` command in our terminal:
```bash
$ go test
```
After running the command, Go will execute all the test functions within our package and report the results.
## Test Coverage
The Go testing framework provides a powerful feature called test coverage, which measures the percentage of code covered by tests. To generate the test coverage report, we can use the `-cover` flag with the `go test` command:
```bash
$ go test -cover
```
By analyzing the coverage report, we can identify untested code blocks and ensure that our tests cover all possible scenarios.
## Benchmarking Tests
Go also provides built-in support for benchmarking tests. These tests help measure the performance of our code by running a given function multiple times and reporting the average execution time.
To write a benchmark test, we need to create a new test function starting with the prefix "Benchmark" followed by the name of the function we want to benchmark. For example, to benchmark our factorial function, we can create the following test function:
```go
func BenchmarkFactorial(b *testing.B) {
for i := 0; i < b.n;="" i++="" {="" factorial(10)="" }="" }="" ```="" to="" run="" the="" benchmark="" tests,="" we="" can="" use="" the="" `go="" test`="" command="" with="" the="" `-bench`="" flag:="" ```bash="" $="" go="" test="" -bench="." ```="" the="" output="" will="" include="" the="" execution="" time="" for="" each="" benchmarked="" function.="" ##="" conclusion="" writing="" tests="" is="" crucial="" for="" ensuring="" the="" reliability="" and="" correctness="" of="" our="" code.="" in="" this="" article,="" we="" explored="" an="" example="" of="" how="" to="" write="" tests="" in="" golang="" using="" the="" built-in="" testing="" package.="" we="" learned="" how="" to="" write="" test="" functions,="" run="" tests,="" analyze="" test="" coverage,="" and="" perform="" benchmarking="" tests.="" by="" following="" these="" practices,="" we="" can="" improve="" the="" quality="" and="" stability="" of="" our="" go="" applications.="">
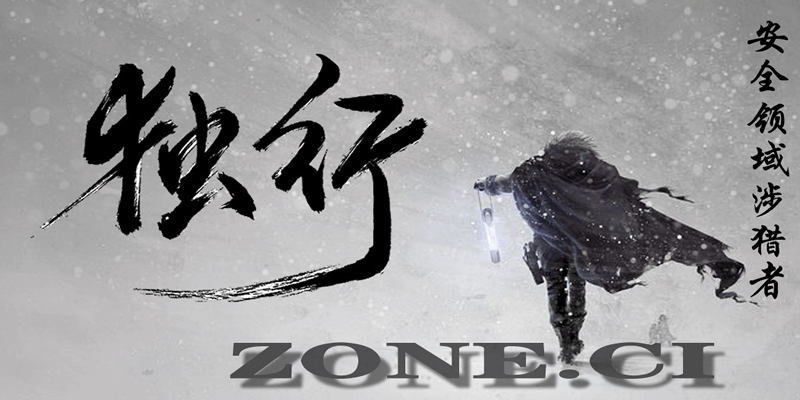
版权声明
本站原创文章转载请注明文章出处及链接,谢谢合作!
评论